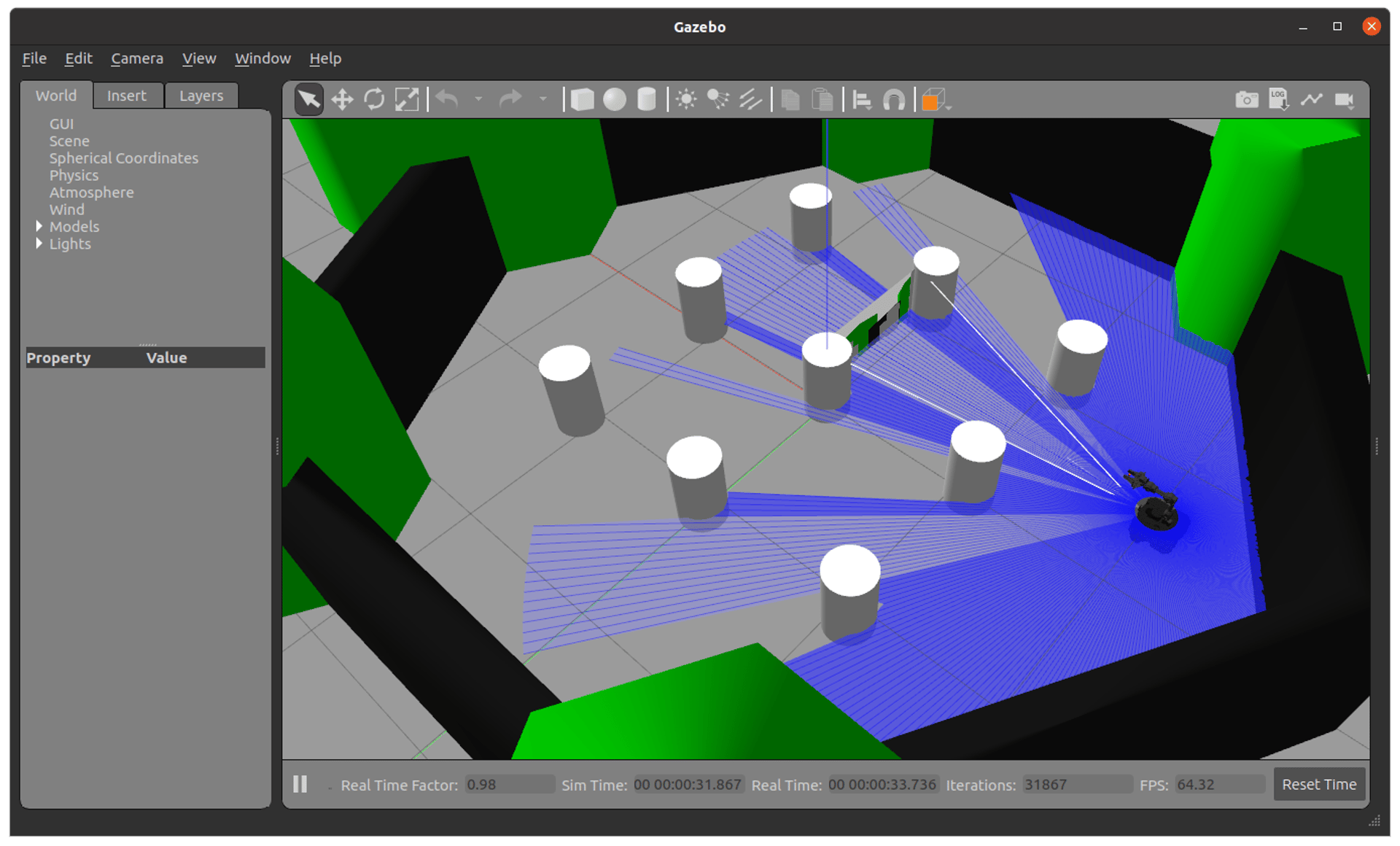
Robotic Operating System
1. Create a package in src:
ros2 pkg create --build-type ament_python pkg_name --dependencies rclpy std_msgs sensor_msgs etc.
• Build package in ros2_ws:
- Individual package build: colcon build --packages-select pkg_name
- Complete build: colcon build (not recommended for our classes)
• Source after opening new terminal window and after new build:
- In /home/ros2: source ./source (check that you are in /home/ros2)
- In any other folders: source /home/ros2/ros2_ws/install/setup.bash
- If ros2 cannot be found after source: /opt/ros/humble/setup.bash
2. Starting nodes:
ros2 run pkg_name executable_name
ros2 launch pkg_name launch_name.launch.py
3. Create a package for own interfaces - custom_interfaces (msg, srv, action)
• ros2 pkg create --build-type ament_cmake custom_interfaces --dependencies rclcpp std_msgs
• inside create folders: msg, srv, action
• modify CMakeList.txt:
Function find_package():
find_package(rosidl_default_generators REQUIRED)
Function rosidl_generate_interfaces():
rosidl_generate_interfaces(${PROJECT_NAME}
"msg/MsgInterfaceName.msg"
)
• modify Package.xml:
<build_depend>rosidl_default_generators</build_depend>
<exec_depend>rosidl_default_runtime</exec_depend>
<member_of_group>rosidl_interface_packages</member_of_group>
ros2 topic (list, …)
ros2 node (list, …)
ros2 service (list, …)
ros2 action (list, …)
ros2 interface (list, …)
4. To add custom_interfaces to your own package
• In package.xml of own package:
• <depend>custom_interfaces</depend
ROS Task #3
ROS #4
ROS #5
The problem statement addresses several tasks within the realm of Robot Operating System (ROS) development. Initially, there's a requirement to craft a launch file capable of initializing a publisher node, with the added specification of commencing data transmission from a particular letter of the alphabet. This necessitates adapting the publisher code accordingly to meet the specified criteria. Additionally, another launch file must be devised to activate a node precisely at a predetermined time, accompanied by a fallback message in the event that the temporal condition is not satisfied. Following this, the creation of a message interface tailored for object classification purposes is outlined, drawing insights from established message interfaces such as vision_msgs to inform its design. Finally, a publisher-subscriber architecture must be implemented to facilitate the navigation of a robot to a designated location marked by a red X within a simulated environment. This entails the creation of a publisher responsible for issuing movement commands to the robot via the /cmd_vel topic, alongside a subscriber tasked with acquiring data from the /odom topic to inform trajectory adjustments necessary for accurate navigation.
1: Create a Launch File for Publisher Initialization:
Develop a launch file to initialize a ROS node for publishing data.
Modify the publisher code to begin transmitting data starting from a specific letter of the alphabet.
2: Design a Launch File for Time-based Node Activation:
Craft a launch file capable of activating a ROS node at a predetermined time.
Implement a mechanism to print a fallback message if the time condition isn't met.
3:Define a Message Interface for Object Classification:
Create a custom message interface tailored for object classification.
Consider fields relevant for object identification, drawing inspiration from existing interfaces like vision_msgs.
4: Implement Publisher-Subscriber System for Robot Navigation:
Launch the turtlebot3 simulation environment using turtlebot3_gazebo.
Create a publisher node to issue movement commands to the robot via the /cmd_vel topic.
Develop a subscriber node to read data from the /odom topic, providing odometry information.
Utilize odometry data to determine trajectory adjustments necessary for navigating the robot to a square marked with a red X.
The task at hand involves creating and utilizing a service interface within a ROS (Robot Operating System) framework to facilitate controlled movement of a robot in a simulated environment. Initially, a service interface named Turn.srv is to be designed, capable of receiving requests for three specific movements: "Turn Right," "Turn Left," and "Stop," with responses indicating the success status of the requested action. Subsequently, a service is implemented to interpret these requests and execute corresponding movements of the robot, thereby enabling dynamic control over its trajectory. Furthermore, the service is invoked programmatically to command the robot's actions. Additionally, manual control is demonstrated through the use of ros2 topic pub, allowing users to manually guide the robot towards a predefined square marked with a red cross sign. To capture relevant data during this movement, a bag process is initiated beforehand to record necessary topics. After reloading the simulation environment using a dedicated service, playback of the recorded bag file is initiated to simulate the robot's navigation towards the designated square while ensuring the capture of essential data for subsequent analysis or playback. Through these steps, a comprehensive framework for controlling and simulating robot movements within ROS is established, demonstrating the versatility and functionality of service-based communication in robotics applications.
2: Create a service interface
a. Create an interface that receives as a request three possible movements: "Turn Right" (turn right), "Turn Left" (turn left) and "Stop" (stop). As a response, it should send you the success status (True/False). The name of the interface should be Turn.srv.
1: Start the Gazebo Simulation
3: Call the service
4: Manually with ros2 topic pub:
a. move the robot to the square with a red cross sign. In the second window start the bag process to record the necessary topics (should be started before the movement)
b. reload the simulation with a service dedicated to the purpose
c. start recorded bag file ( X: 1.00 , Y: -5.00 )
In this problem, we're essentially designing a system to control a robot's movement towards a wall in a precise manner. To achieve this, we're going to create an action interface and server within the ROS environment.
Firstly, we need to define an action interface, which essentially outlines the plan or goal for the robot's movement. In this case, the goal is for the robot to stop at a certain distance from the wall. We'll also include feedback, which will provide updates on the progress of the movement. This feedback will be represented by the number of seconds the robot has been moving.
Next, we'll develop an action server. This server will be responsible for executing the plan outlined in the action interface. It will control the robot's movement towards the wall, making sure to stop it at the specified distance. To do this effectively, the server will utilize data from a LiDAR sensor, which will help determine the robot's distance from the wall in real-time.
1: Start the Gazebo Simulation & Adjust Robot to the Coordinate at ( X: 0.00 , Y: -4.00 )
2: Create an action interface:
a. Goal: meters to stop in front of the wall. Feedback – number of seconds the robot moves
3: Create an action server with a newly generated action interface:
a. Move to the wall and stop in front of it so the LiDAR identify the meters left and compare with the goal
4: Sending Goal Directly Via Terminal: